标签:buffer ogr 类型 address add ide tps spl http
和其他的库一样,每个库都可能有自己独特的数据结构,例如OpenCV,numpy库的多维数组叫做ndarray( N dimensionality array ),它的内存结构如下图: ndarray的内存结构 在这个结构体中有两个对象,一个是用来描述元素类型的头部区域,一个是用来储存数据的数据区域。(事实上大多数数据类型的数据都是这么储存的)。
2 ndarray对象的创建
2.1 ndarray多维数组的创建常规方法 创建一个3*3的数组并在屏幕打印它以及它的类型和维数:
import numpy as np
x = np.array([[0,1,2],[3,4,5],[6,7,8]],dtype = np.int32)
print(‘这个数组是:‘,x)
print(‘这个数组的数据类型是:‘,x.dtype)
print(‘这个数组的大小:‘,x.shape)
[/code]
?
屏幕输出结果:
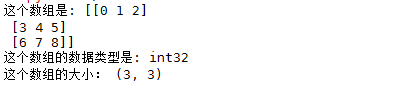
?
我们也可以采用更加直接的办法:
?
```code
import numpy as np
x = np.arange(0,9).reshape(3,3)
print(‘这个数组是:‘,x)
print(‘这个数组的数据类型是:‘,x.dtype)
print(‘这个数组的大小:‘,x.shape)
[/code]
?
屏幕上打印输出的结果和前一种的结果是一样的。
?
2.2 ndarray多维数组的创建其他方法
除了常规方法,numpy还提供了一些其他的创建方法:
2.2.1 创建全0或者全1的数组
?
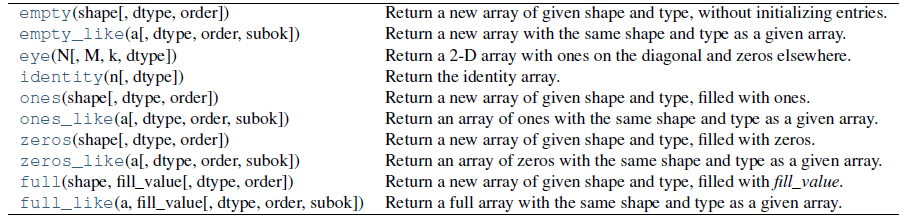
例如:
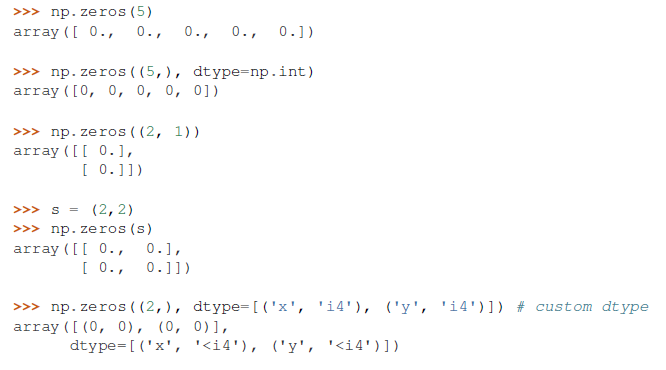
?
```code
import numpy as np
x = np.ones([3,3])
print(‘这个数组是:‘,x)
print(‘这个数组的数据类型是:‘,x.dtype)
print(‘这个数组的大小:‘,x.shape)
[/code]
?
```code
>>> x = np.arange(6)
>>> x = x.reshape(2,3)
>>> x
array([[0, 1, 2], [3, 4, 5]])
>>> np.ones_like(x)
array([[1, 1, 1], [1, 1, 1]])
>>> y = np.arange(3, dtype=np.float)
>>> y
array([ 0., 1., 2.])
>>> np.ones_like(y)
array([ 1., 1., 1.])
[/code]
?
当然也可以填充其他的数:
?
```code
import numpy as np
x = np.full([3,3],np.inf)
print(‘这个数组是:‘,x)
print(‘这个数组的数据类型是:‘,x.dtype)
print(‘这个数组的大小:‘,x.shape)
[/code]
?
打印输出: 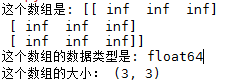
?
2.2.2 从已存在的数据中创建数组
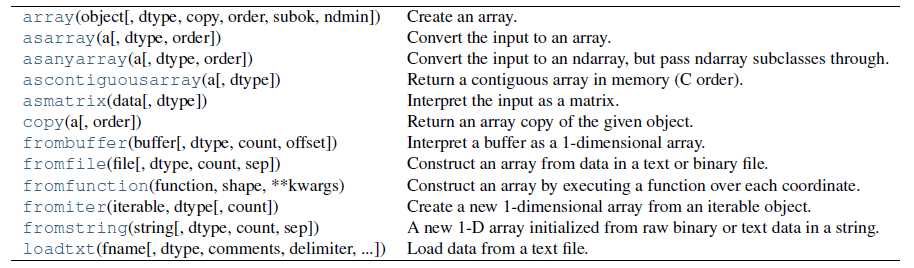
?
```code
>>> np.array([1, 2, 3])
array([1, 2, 3])
>>> np.array([1, 2, 3.0])
array([ 1., 2., 3.])
>>> np.array([[1, 2], [3, 4]])
array([[1, 2], [3, 4]])
>>> np.array([1, 2, 3], ndmin=2)
array([[1, 2, 3]])
>>> np.array([1, 2, 3], dtype=complex)
array([ 1.+0.j, 2.+0.j, 3.+0.j])
>>> x = np.array([(1,2),(3,4)],dtype=[(‘a‘,‘<i4‘),(‘b‘,‘<i4‘)])
>>> x[‘a‘]
array([1, 3])
[/code]
?
2.2.3 创建记录阵列(record array,可能翻译不准):创建一个阵列,将其他数组集中在一起管理,并可以命名:

例如:
?
```code
import numpy as np
x1 = np.arange(0,3)
x2 = np.array([‘ff‘,‘hh‘,‘yy‘])
x3 = ([1,2,3])
r = np.core.records.fromarrays([x1,x2,x3],names=‘a,b,c‘)
print(r[2])
print(r.a)
[/code]
?
2.2.4 创建字符数组
numpy提供了专门的函数创建字符数组:np.chararray()
首先看看它的参数:
Parameters
| ———-
| shape : tuple
| Shape of the array.
| itemsize : int, optional
| Length of each array element, in number of characters. Default is 1.
| unicode : bool, optional
| Are the array elements of type unicode (True) or string (False).
| Default is False.
| buffer : int, optional
| Memory address of the start of the array data. Default is None,
| in which case a new array is created.
| offset : int, optional
| Fixed stride displacement from the beginning of an axis?
| Default is 0. Needs to be >=0.
| strides : array_like of ints, optional
| Strides for the array (see ndarray.strides for full description).
| Default is None.
| order : {‘C’, ‘F’}, optional
| The order in which the array data is stored in memory: ‘C’ ->
| “row major” order (the default), ‘F’ -> “column major”
| (Fortran) order.
?
```code
Examples
| --------
| >>> charar = np.chararray((3, 3))
| >>> charar[:] = ‘a‘
| >>> charar
| chararray([[‘a‘, ‘a‘, ‘a‘],
| [‘a‘, ‘a‘, ‘a‘],
| [‘a‘, ‘a‘, ‘a‘]],
| dtype=‘|S1‘)
|
| >>> charar = np.chararray(charar.shape, itemsize=5)
| >>> charar[:] = ‘abc‘
| >>> charar
| chararray([[‘abc‘, ‘abc‘, ‘abc‘],
| [‘abc‘, ‘abc‘, ‘abc‘],
| [‘abc‘, ‘abc‘, ‘abc‘]],
| dtype=‘|S5‘)
[/code]
?
2.2.5 创建数值数组

?
```code
import numpy as np
x1 = np.arange(0,3,1)
x2 = np.linspace(0,3,4)
x3 = np.logspace(1,8,3)
x4 = np.mgrid[0:3,0:3]
x5 = np.ogrid[0:3,0:3]
print(x1,x2,x3,x4,x5)
[/code]
?
2.2.6 创建矩阵

?
2.2.7 矩阵类(The matrix class)

?
?
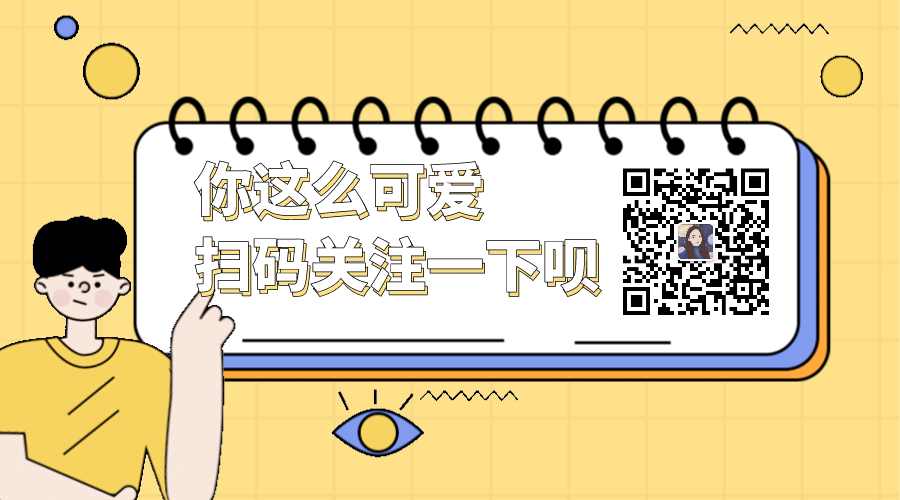
标签:buffer ogr 类型 address add ide tps spl http
原文地址:https://www.cnblogs.com/gc2770/p/14960706.html