2.在application.properties配置文件中添加数据库的支持
- #DB Configuration:
- spring.datasource.driverClassName = com.mysql.jdbc.Driver
- spring.datasource.url = jdbc:mysql://localhost:3306/mybatis_db?useUnicode=true&characterEncoding=utf-8&useSSL=false
- spring.datasource.username = root
- spring.datasource.password = 220316
这个关于数据库配置的代码其实很简单
第一个是数据库所用的驱动
第二个是数据库url地址,这里我连接的名字为mybatis_db数据库
第三第四是数据库连接的用户名和密码
3.pom.xml文件中添加相应的jar包
这里我就不一一介绍了,大家看一下demo就知道需要添加哪些内容了。
主要是SpringBoot相关的jar包,mybatis相关的jar包就ok了
- SET FOREIGN_KEY_CHECKS=0;
- DROP TABLE IF EXISTS `user`;
- CREATE TABLE `user` (
- `user_id` int(10) NOT NULL AUTO_INCREMENT,
- `user_code` varchar(20) DEFAULT NULL,
- `user_name` varchar(20) DEFAULT NULL,
- `nick_name` varchar(20) DEFAULT NULL,
- `user_pwd` varchar(20) DEFAULT NULL,
- `create_date` datetime DEFAULT NULL,
- `update_date` datetime DEFAULT NULL,
- PRIMARY KEY (`user_id`)
- ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;
- INSERT INTO `user` VALUES (‘1‘, ‘10001‘, ‘user10001‘, ‘no1‘, ‘123456‘, ‘2017-10-22 15:23:32‘, ‘2017-10-22 15:23:35‘);
- INSERT INTO `user` VALUES (‘2‘, ‘10002‘, ‘user10002‘, ‘no2‘, ‘123456‘, ‘2017-10-22 15:23:32‘, ‘2017-10-22 15:23:35‘);
6.接下来就是最重要的编写Mapper接口,我们这里采用通过注解来实现数据库的增删改查功能。
增删改查分别对应的注解为@Insert,@Delete,@Update,@Select
关于注解的使用细节,我这里举select的例子来给大家解释一下。
- @Select("select * from user")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- public List<UserEntity> queryList();
上面是查询数据库user表的所有数据,然后会自动的转为list集合。其中column里面的字段名称必须和数据库里面表的字段一模一样,property里面的字段属性必须和UserEntity这个实体类对应的字段一模一样。
- @Select("SELECT * FROM USER WHERE user_id = #{userId}")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- UserEntity findById(long userId);
上面这种形式是通过传入一个字段来查询数据。这里需要注意的是#{}里面的字段内容必须和findById里面传入的字段一模一样。除了#{}以外的字段都必须满足sql的查询格式。这里大家不要误解了,findById可以随便定义和jpa中是不一样的。
下面将给出增删改查的所有代码,如下所示:
- package com.joy.dao;
- import java.util.List;
- import java.util.Map;
- import org.apache.ibatis.annotations.*;
- import com.joy.entity.UserEntity;
- public interface UserMapper {
- @Select("select * from user")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- public List<UserEntity> queryList();
- @Select("SELECT * FROM USER WHERE user_id = #{userId}")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- UserEntity findById(long userId);
-
- @Insert("INSERT INTO USER(nick_name, user_code) VALUES(#{nickName}, #{userCode})")
- int insertParam(@Param("nickName") String nickName, @Param("userCode") String userCode);
-
- @Insert("INSERT INTO USER(nick_name, user_code) VALUES(#{nickName,jdbcType=VARCHAR}, #{userCode,jdbcType=INTEGER})")
- int insertByMap(Map<String, Object> map);
-
- @Insert("insert into user(nick_name,user_code,user_name,user_pwd,create_date,update_date) values(#{nickName},#{userCode},#{userName},#{userPwd},#{createDate},#{updateDate})")
- public int insertEntity(UserEntity entity);
-
- @Update("UPDATE user SET nick_name=#{nickName} WHERE user_id=#{userId}")
- int updateEntity(UserEntity user);
-
- @Delete("DELETE FROM user WHERE user_id =#{userId}")
- int delete(Long userId);
-
- @Delete("DELETE FROM user WHERE user_id =#{userId}")
- int deleteEntity(UserEntity entity);
- }
7.最后就是编写相应的service和controller类来调用这些增删改查的接口。
service类信息:
- package com.joy.service;
- import java.util.Date;
- import java.util.HashMap;
- import java.util.List;
- import java.util.Map;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.stereotype.Service;
- import com.joy.dao.UserMapper;
- import com.joy.entity.UserEntity;
- @Service
- public class UserService {
- @Autowired(required = false)
- private UserMapper mapper;
-
- public List<UserEntity> queryList(){
- List<UserEntity> userList=mapper.queryList();
- return userList;
- }
-
- public UserEntity findById(long userId){
- System.out.println("userId:"+userId);
- return mapper.findById(userId);
- }
-
- public int insertEntity() {
- UserEntity entity=new UserEntity();
- entity.setUserName("lisi");
- entity.setUserCode("lisi"+new Date());
- entity.setNickName("郭靖");
- entity.setUserPwd("123");
- entity.setCreateDate(new Date());
- entity.setUpdateDate(new Date());
- return mapper.insertEntity(entity);
- }
-
- public int insertParam() {
- return mapper.insertParam("linzhiqiang","lzq");
- }
-
- public int insertByMap() {
- Map<String, Object> map=new HashMap<String, Object>();
- map.put("nickName","zhaotong");
- map.put("userCode","zt");
- return mapper.insertByMap(map);
- }
-
- public int updateEntity() {
- UserEntity entity=new UserEntity();
- entity.setUserId(1);
- entity.setNickName("郭靖");
- return mapper.updateEntity(entity);
- }
-
- public int deleteEntity() {
- UserEntity entity=new UserEntity();
- entity.setUserId(11);
- return mapper.deleteEntity(entity);
- }
- }
controller类信息:
- package com.joy.controller;
- import java.util.List;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RestController;
- import com.github.pagehelper.PageHelper;
- import com.joy.entity.UserEntity;
- import com.joy.service.UserService;
- @RestController
- public class UserController {
- @Autowired
- private UserService userService;
- @RequestMapping("/userlist")
- public List<UserEntity> queryList(){
- PageHelper.startPage(1, 2);
- return userService.queryList();
- }
-
- @RequestMapping("/queryUser")
- public UserEntity queryUserEntity(long userId){
- UserEntity userEntity=userService.findById(userId);
- return userEntity;
- }
-
- @RequestMapping("/insert")
- public int insertEntity() {
- return userService.insertEntity();
- }
-
- @RequestMapping("/insertParam")
- public int insertParam() {
- return userService.insertParam();
- }
-
- @RequestMapping("/insertByMap")
- public int insertByMap() {
- return userService.insertByMap();
- }
-
- @RequestMapping("/updateEntity")
- public int updateEntity() {
- return userService.updateEntity();
- }
-
- @RequestMapping("/deleteEntity")
- public int deleteEntity() {
- return userService.deleteEntity();
- }
- }
8.执行结果如下所示:

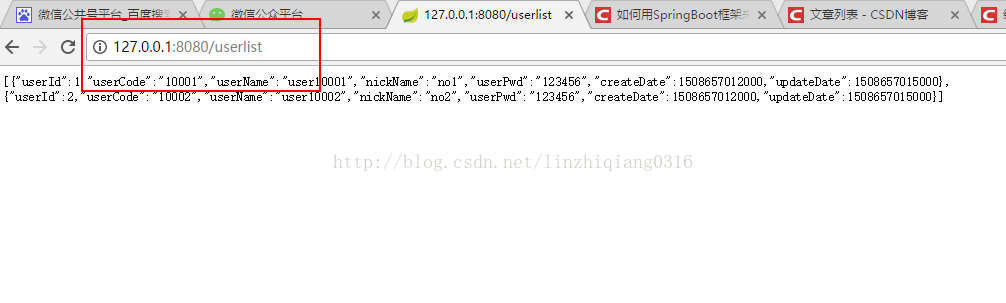
8.如果想要将执行的sql打印在控制台上面,可以在application.properties添加如下的配置信息。
logging.level.com.joy=DEBUG
这里需要特别注意的是:level后面是你项目包的地址不要写的和我一样。
到这里关于SpringBoot整合Mybatis项目就介绍完毕,如果大家想要源代码的话可以去我的GitHub地址下载。
如果按照博客上面写的步骤操作还有问题,可以观看我为大家录制的视频讲解。因为本人不是专业的讲师,所以可能讲的不是特别细致。有什么建议都可以提出来,谢谢大家。视频地址:https://pan.baidu.com/s/1qYcFDow
点击打开链接
如果大家对文章有什么问题或者疑意之类的,可以加我订阅号在上面留言,订阅号上面我会定期更新最新博客。如果嫌麻烦可以直接加我wechat:lzqcode