标签:rate package 单例 void start 结果 not imp 掌握
什么是工厂模式?
工厂模式(Factory Pattern)在实际开发中非常常见,它可以由用户决定创建对象的类型。
因为工厂模式,我们无需向客户暴露我们的代码逻辑,只需要开放接口给客户,由客户指定要创建的对象的类型,便可以动态生成符合需求的类别对象。
什么场合用工厂模式?
需要根据实际情况才能决定创建何种类别对象的场景。
听起来挺悬乎的,其实很容易理解。
假设,你要从广州到深圳,你就需要交通工具。
选择可就多了,有大巴,有火车,有高铁,有动车,未来还有地铁和城轨。
你在不同时刻有不同的选择。
比较,你是土豪,你还可以坐飞机。
你不想转车,可能直接大巴。
你要便宜点,你可以坐火车。
干净舒适时,你可以选择高铁。
下面,实例讲解。
工厂模式的实现手段
交通工具可以是一个抽奖类,也可以是一个接口。
所以,我们定义一个接口代表交通工具,之后把大巴、火车、飞机实现这个接口,UML 图如下。
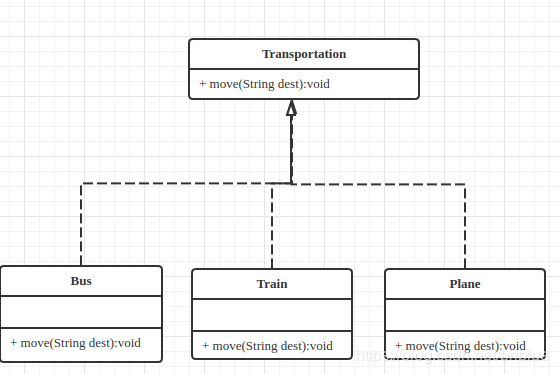
我们再编写工厂类。
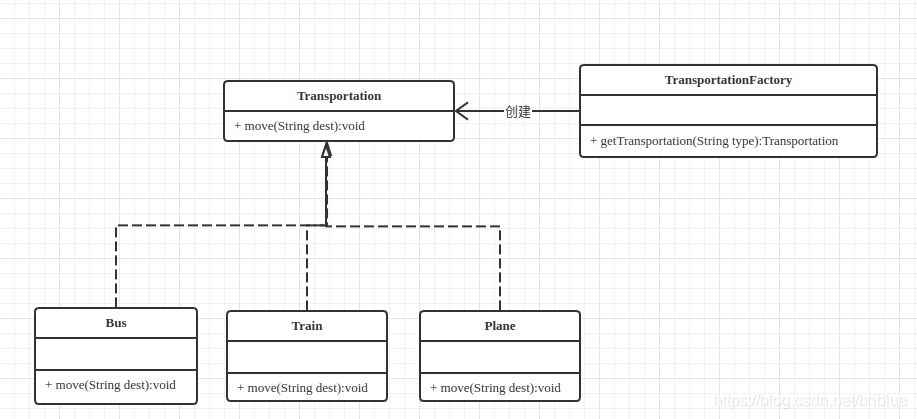
我们通过 TransportationFactory 这个类中的 getTransportation() 方法就可以创建类了。
下面是 Java 代码示意:
Transportation.java
- public interface Transportation {
- void move(String dst);
- }
再实现 Bus、Train、Plane
Bus.java
- public class Bus implements Transportation{
-
- @Override
- public void move(String dst) {
- System.out.println("Take a bus to "+dst);
- }
- }
Train.java
- public class Train implements Transportation{
-
- @Override
- public void move(String dst) {
- System.out.println("Take a train to "+dst);
- }
- }
Plane.java
- public class Plane implements Transportation{
-
- @Override
- public void move(String dst) {
- System.out.println("Take a plane to "+dst);
- }
- }
然后,我们实现工厂类
Transportation.java
- public class TranportationFactory {
-
- public Transportation getTransportation(String type){
- if(type == null){
- return null;
- }
- if(type.equalsIgnoreCase("TRAIN")){
- return new Train();
- } else if(type.equalsIgnoreCase("BUS")){
- return new Bus();
- } else if(type.equalsIgnoreCase("PLANE")){
- return new Plane();
- }
- return null;
- }
-
- }
可以看到,工厂类根据 type 的不同,选择创建不同的类别对象。
最后,编写测试代码。
FactoryDemo.java
- public class FactoryDemo {
-
- public static void main(String[] args) {
-
- TranportationFactory factory = new TranportationFactory();
-
- Transportation transportation = factory.getTransportation("train");
-
- transportation.move("深圳");
- }
-
- }
最终,打印结果如下:
Take a train to 深圳
我们可以看到,通过简单的例子,我们掌握了工厂模式的基础方法,下面通过实际的源码例子加深理解。
Android 源码中的工厂模式
xref: /packages/apps/Camera2/src/com/android/camera/debug/Logger.java
- public interface Logger {
-
- ......
-
- public interface Factory {
- public Logger create(Tag tag);
- }
- }
这是 Android 系统应用相机的一份代码,主要是记录 Log 的,但它是一个接口,说明这个应用中有不同的 Logger,并且里面还有一个接口 Factory,通过传入 Tag 可以生成不同的 Logger。
经查询 Logger 有 2 种实现类,TagLogger 和 NoOpLogger,它们的代码在 Loggers.java 中。
因为篇幅所限,这里只讨论 TagLogger。
xref: /packages/apps/Camera2/src/com/android/camera/debug/Loggers.java
- public class Loggers {
-
- public static Logger.Factory tagFactory() {
- return TagLoggerFactory.instance();
- }
-
- public static Logger.Factory factoryFor(final Logger logger) {
- return new Logger.Factory() {
- @Override
- public Logger create(Tag tag) {
- return logger;
- }
- };
- }
-
- private static class TagLoggerFactory implements Logger.Factory {
- private static class Singleton {
- private static final TagLoggerFactory INSTANCE = new TagLoggerFactory();
- }
-
- public static TagLoggerFactory instance() {
- return Singleton.INSTANCE;
- }
-
- @Override
- public Logger create(Tag tag) {
- return new TagLogger(tag);
- }
- }
-
- private static class TagLogger implements Logger {
- private final Log.Tag mTag;
-
- public TagLogger(Log.Tag tag) {
- mTag = tag;
- }
- ...
-
- }
- }
很惊喜的是,这个例子也可以用来解释单例模式。
TagLogger.Factory 中的 create 方法可以创建 TaggLogger。
所以,从入口方法
factoryFor()
就可以决定不同的 Factory,不同的 Factory 就创建了不同的 Logger。
这显然是工厂模式。
设计模式(二)
标签:rate package 单例 void start 结果 not imp 掌握
原文地址:https://www.cnblogs.com/cuimian/p/13940786.html