标签:图片 == john com 自己 声明 大小 描述 char
// 练习:使用二分查找,在一组有序元素中查找数据项
// 形参是数组,实参是数组名
#include<stdio.h>
#include <stdlib.h>
const int N=5;
int binarySearch(int x[], int n, int item); // 函数声明
int main() {
int a[5] = { 2,7,19,45,66 };
int i, index, key;
printf("数组a中的数据:\n");
for (i = 0; i < N; i++)
printf("%d ", a[i]);
printf("\n");
printf("输入待查找的数据项: ");
scanf("%d", &key);
// 调用函数binarySearch()在数组a中查找指定数据项item,并返回查找结果给index
// 补足代码①
index = binarySearch(a,N,key);
if(index>=0)
printf("%d在数组中,下标为%d\n", key, index);
else
printf("%d不在数组中\n", key);
system("pause");
return 0;
}
//函数功能描述:
//使用二分查找算法在数组x中查找特定值item,数组x大小为n
// 如果找到,返回其下标
// 如果没找到,返回-1
int binarySearch(int x[], int n, int item) {
int low, high, mid;
low = 0;
high = n-1;
while(low <= high) {
mid = (low+high)/2;
if (item == x[mid])
return mid;
else if(item == x[low])
high = mid - 1;
else
low = mid + 1;
}
return -1;
}
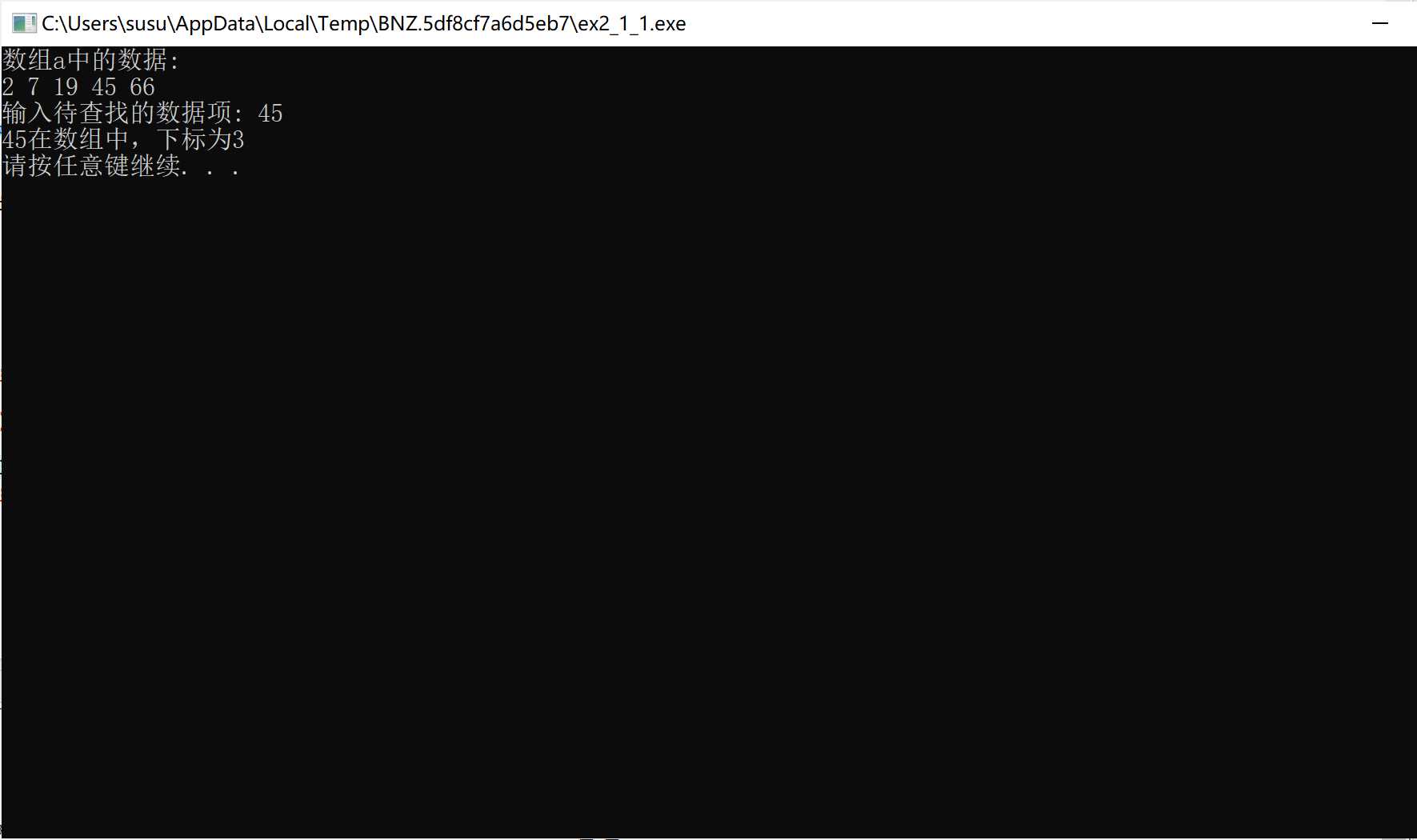
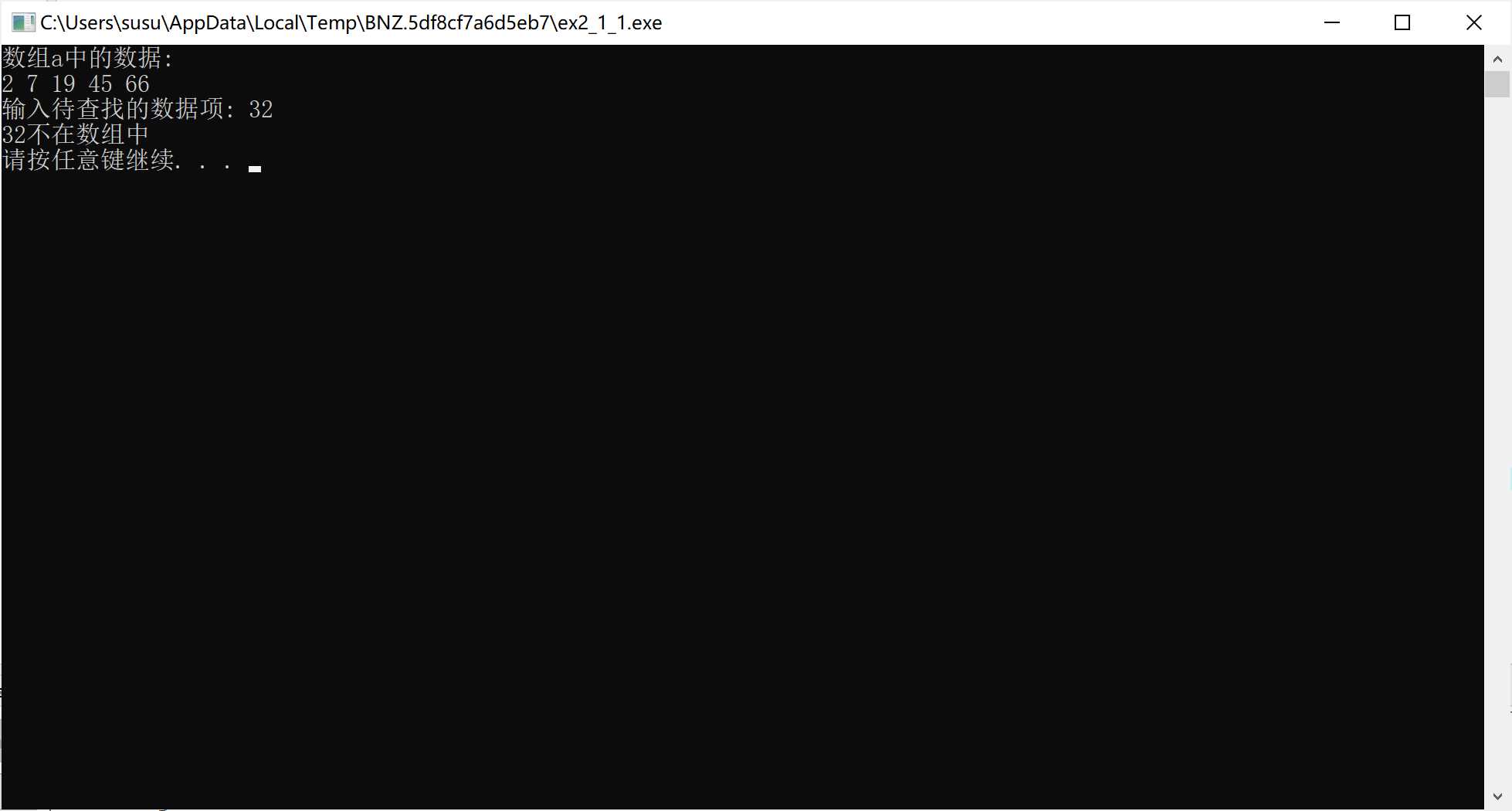
/* N个字典序的整数已放在一维数组中,给定下列程序中,函数fun的功能是: 利用折半查找算法查找整数m在数组中的位置。 若找到,则返回其下标值;反之,则返回-1 */ #include<stdio.h> #include<stdlib.h> #define N 10 int fun(int* a, int m) { int low = 0, high = N - 1, mid; /*************ERROR**************/ while (low <= high) { mid = (low + high) / 2; /*************ERROR**************/ if (m < *(a + mid)) high = mid - 1; /*************ERROR**************/ else if (m > * (a + mid)) low = mid + 1; else return(mid); } return(-1); } int main() { int i, a[N] = { -3,4,7,9,13,24,67,89,100,180 }, k, m; printf("a数组中的数据如下:\n"); for (i = 0; i < N; i++) printf("%d ", a[i]); printf("\nEnter m: \n"); scanf("%d", &m); /*************ERROR**************/ k = fun(a, m); if (k >= 0) printf("m=%d,index=%d\n", m, k); else printf("Not be found!\n"); system("pause"); return 0 }
// 练习:使用选择法对字符串按字典序排序 #include <stdio.h> #include <string.h> #include <stdlib.h> void selectSort(char str[][20], int n ); // 函数声明,形参str是二维数组名 int main() { char name[][20] = {"John", "Alex", "Joseph", "Taylor", "George"}; int i; printf("输出初始名单:\n"); for (i = 0; i < 5; i++) printf("%s\n", name[i]); selectSort(name, 5); // 调用选择法对name数组中的字符串排序 printf("按字典序输出名单:\n"); for(i=0; i<5; i++) printf("%s\n", name[i]); system("pause"); return 0; } // 函数定义 // 函数功能描述:使用选择法对二维数组str中的n个字符串按字典序排序 void selectSort(char str[][20], int n) { int i,j,k; char temp[20]; for(i=0;i<n-1;i++) { k=i; for(j=1+i;j<n;j++) { if(strcmp(str[j],str[k])<0) k=j; } if(k!=i) strcpy(temp,str[i]); strcpy(str[i],str[k]); strcpy(str[k],temp); } }
/* 假定输入的字符串中只包含字母和*号。 编写函数,实现: 除了字符串前导的*号之外,将串中其他*号全部删除。 在编写函数时,不得使用C语言提供的字符串函数。 例如,若字符串中的内容为****A*BC*DEF*G******* 删除后,字符串中的内容则应当是****ABCDEFG */ #include <string.h> #include <stdio.h> #include <stdlib.h> void fun(char *a) { /*****ERROR********/ int i=0; char *p = a; /****ERROR***/ while(*p && *p == ‘*‘) { a[i] = *p; i++; p++; } while(*p) { /******ERROR*******/ if(*p != ‘*‘) { a[i] = *p; i++; } p++; } /******ERROR*******/ a[i] = ‘\0‘; } int main() { char s[81]; printf("Enter a string :\n"); gets(s); /***ERROR******/ fun(&s[0]); printf("The string after deleted:\n"); puts(s); system("pause"); return 0; }
/* 假定输入的字符串中只包含字母和*号。 编写函数,实现: 除了字符串前导和尾部的*号之外,将串中其他*号全部删除。 在编写函数时,不得使用C语言提供的字符串函数。 例如,若字符串中的内容为****A*BC*DEF*G******* 删除后,字符串中的内容则应当是****ABCDEFG****** */ #include <stdio.h> #include <stdlib.h> #include <string.h> void fun(char *a) { /**ERROR******/ int i=0; char *t = a, *f = a; char *q = a; while(*t) t++; t--; while(*t == ‘*‘) t--; while(*f == ‘*‘) f++; /***ERROR***/ while (q<f) { a[i] = *q; q++; i++; } while (q<t) { /***ERROR**/ if(*q != ‘*‘) { a[i] = *q; i++; } q++; } while (*q) { a[i] = *q; i++; q++; } /**ERROR**/ a[i]=‘\0‘; } int main () { char s[81]; printf("Entre a string:\n"); gets(s); /**ERROR**/ fun(& s[0]); printf("The sting after deleted:\n"); puts(s); system("pause"); return 0; }
最后两个改错好像差不多?就第二个错误处有些不一样,其余错误也是十分基础的细节型错误,只要前面章节学的不是太差应该都可以很轻松的找出来,况且老师还标注了错误位置。
关于数组与指针,在实验方面的运用实在是有些生疏,而且在思维上的要求也比较高,看老师给的带注释的程序源码文件就比较容易看懂,自己写的时候完全就是一头雾水,还需加强巩固。
标签:图片 == john com 自己 声明 大小 描述 char
原文地址:https://www.cnblogs.com/sdlad/p/12057128.html